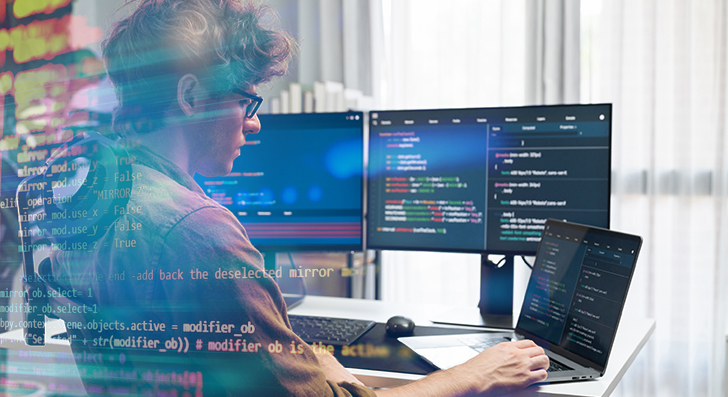
Scalability signifies your software can cope with expansion—a lot more customers, extra facts, plus much more targeted traffic—without having breaking. Being a developer, creating with scalability in your mind saves time and stress afterwards. Right here’s a transparent and functional manual to assist you start by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't one thing you bolt on afterwards—it should be aspect of one's approach from the start. Numerous purposes fail if they develop speedy since the first style can’t deal with the additional load. As a developer, you must Imagine early about how your process will behave under pressure.
Commence by building your architecture for being versatile. Stay clear of monolithic codebases in which anything is tightly connected. As an alternative, use modular structure or microservices. These patterns break your application into smaller sized, impartial pieces. Every module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your databases from working day a person. Will it require to deal with 1,000,000 users or simply a hundred? Select the proper form—relational or NoSQL—dependant on how your information will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial issue is to avoid hardcoding assumptions. Don’t create code that only functions beneath recent problems. Contemplate what would materialize In the event your person base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven techniques. These aid your application take care of far more requests with no receiving overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you might be cutting down long run complications. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s much better to arrange early than to rebuild later.
Use the ideal Databases
Selecting the right databases can be a crucial part of setting up scalable apps. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or simply lead to failures as your app grows.
Start out by comprehension your information. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. Additionally they assistance scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
In case your facts is more versatile—like user exercise logs, item catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more conveniently.
Also, contemplate your examine and write designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a large produce load? Look into databases that will cope with superior create throughput, as well as celebration-centered information storage techniques like Apache Kafka (for momentary information streams).
It’s also wise to Consider in advance. You might not have to have Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t have to have to change later on.
Use indexing to speed up queries. Keep away from unneeded joins. Normalize or denormalize your facts based upon your obtain styles. And normally monitor databases efficiency while you expand.
In a nutshell, the best database is determined by your app’s construction, speed requirements, and how you expect it to grow. Get time to pick wisely—it’ll save a lot of hassle afterwards.
Enhance Code and Queries
Rapidly code is key to scalability. As your app grows, each individual compact hold off provides up. Inadequately composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by producing clean up, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complex Alternative if an easy a single works. Keep the features limited, targeted, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes way too lengthy to operate or employs an excessive amount of memory.
Subsequent, evaluate your databases queries. These often sluggish issues down much more than the code by itself. Make sure Just about every query only asks for the information you actually will need. Keep away from Choose *, which fetches anything, and as an alternative find certain fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Specially across massive tables.
If you see the identical facts being requested time and again, use caching. Store the outcome quickly using resources like Redis or Memcached therefore you don’t really have to repeat costly operations.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app additional economical.
Remember to test with huge datasets. Code and queries that operate great with one hundred information may possibly crash every time they have to take care of one million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques enable your software stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more site visitors. If every little thing goes by means of a single server, it is going to immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming website traffic across several servers. As opposed to 1 server carrying out each of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no single server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused quickly. When people request the same facts once again—like a product site or even a profile—you don’t need to fetch it within the database each time. You could provide it from the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information close to the consumer.
Caching minimizes databases load, improves pace, and will make your app extra effective.
Use caching for things which don’t alter generally. And usually be sure your cache is updated when info does modify.
Briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your application deal with additional users, remain rapid, and recover from difficulties. If you intend to mature, you will need both.
Use Cloud and Container Equipment
To develop scalable purposes, you'll need equipment that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capacity. When visitors will increase, it is possible to incorporate additional methods with just a couple clicks or mechanically working with auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You are able to focus on building your application in lieu of taking care of infrastructure.
Containers are A different essential Device. A container packages your application and all the things it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 part of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale pieces independently, that's great for effectiveness and reliability.
Briefly, working with cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, reduce threat, and assist you stay centered on developing, not repairing.
Observe Every thing
In case you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make improved decisions as your app grows. It’s a essential Component of building scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—observe your app too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes transpire, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s going on within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you ought to get notified right away. This aids you repair problems here fast, normally right before people even observe.
Checking can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in errors or slowdowns, you may roll it back again prior to it causes authentic hurt.
As your app grows, targeted visitors and knowledge improve. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct equipment set up, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the proper applications, you'll be able to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create good.